PyFonts: a simple way to load fonts for matplotlib
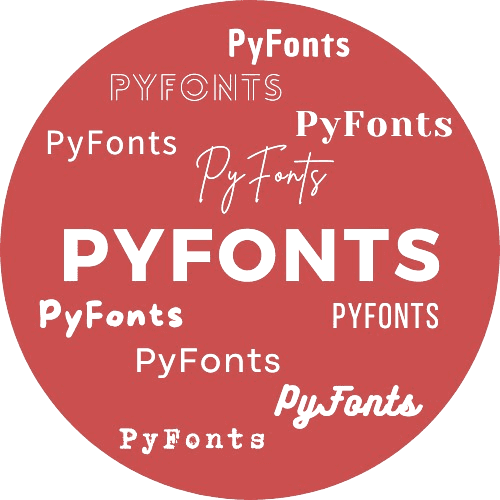
PyFonts is a library that allows to load easily any font from the web and use it in your matplotlib charts.
It was created by Joseph Barbier in order to simplify the process to loading fonts in matplotlib and remove the need to install them on your computer.
⏱ Quick start
Before using pyfonts you need to install it. This can easily be done with pip
:
pip install pyfonts
pyfonts has a main simple function:load_google_font()
: load a font from Google font and return a matplotlib font object.
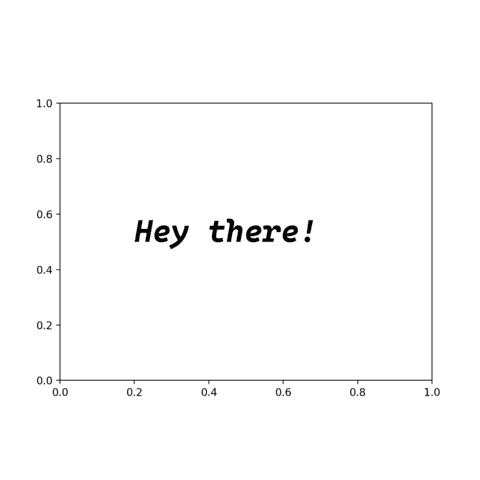
Basic use case of pyfonts
import matplotlib.pyplot as plt
from pyfonts import load_google_font
font = load_google_font("Cascadia Mono", weight="bold", italic=True)
fig, ax = plt.subplots()
ax.text(
x=0.2,
y=0.5,
s="Hey there!",
size=30,
font=font # we pass it to the 'font' argument
)
plt.show()
How to find good fonts
The easiest way to find great is to
- browse Google Font
- copy the name of the font you like
- pass it to
load_google_font("Font Name")
Then you can use the weight
argument to control is the font should be bold or thin for example.
You can control if the font should be italic by specifying italic=True
(default to False
).
Fonts that are not on Google font
Since not all fonts are available on Google fonts, pyfonts
provides a load_font()
function that allows to load a font from any arbitrary url that points to a font file.
Most font files are on Github repositories. In order to load a font from a Github repository, you have to:
- Copy the url of that font file. Here we'll use this url from pyfonts Github repository:
- Then we add
?raw=true
at the end, which gives us:
https://github.com/JosephBARBIERDARNAL/pyfonts/blob/main/tests/Ultra-Regular.ttf
https://github.com/JosephBARBIERDARNAL/pyfonts/blob/main/tests/Ultra-Regular.ttf?raw=true
Use load_font()
by passing the font's URL.
Then, provide the output of load_font()
directly to any matplotlib function that accepts a font, such as ax.text()
.
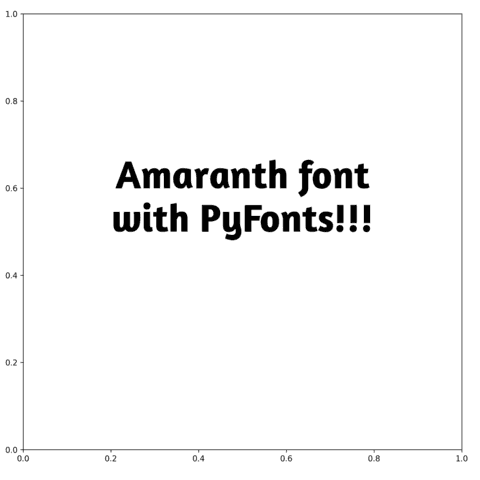
how to load a font with pyfonts
import matplotlib.pyplot as plt
from pyfonts import load_font
font = load_font(
"https://github.com/google/fonts/blob/main/ofl/amaranth/Amaranth-Bold.ttf?raw=true"
)
fig, ax = plt.subplots(figsize=(10, 10), dpi=300)
ax.text(
x=0.5,
y=0.5,
s=f"Amaranth font\nwith PyFonts!!!",
font=font,
fontsize=50,
ha="center",
)
plt.show()
Different weight and style
When you load a font, you don't load all its extensions: bold, italic, thin etc, but only the one from the url. If you want to be able to use a font and its bold version, for example, you need to load both fonts:
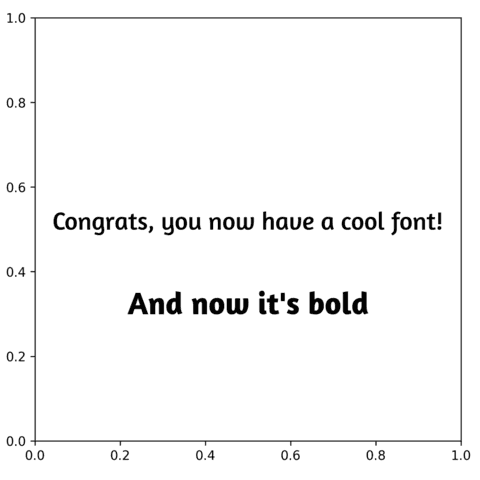
Combine a normal font and a bold font with pyfonts
import matplotlib.pyplot as plt
from pyfonts import load_font
font = load_font(
"https://github.com/google/fonts/blob/main/ofl/amaranth/Amaranth-Regular.ttf?raw=true"
)
bold_font = load_font(
"https://github.com/google/fonts/blob/main/ofl/amaranth/Amaranth-Bold.ttf?raw=true"
)
fig, ax = plt.subplots(figsize=(6, 6), dpi=300)
ax.text(
x=0.5,
y=0.5,
s=f"Congrats, you now have a cool font!",
font=font,
fontsize=20,
ha="center",
)
ax.text(x=0.5, y=0.3, s=f"And now it's bold", font=bold_font, fontsize=25, ha="center")
plt.show()
Locally stored font
PyFonts
also allows you to load a font file that you have on your own computer. You just have to give it the path to your font.
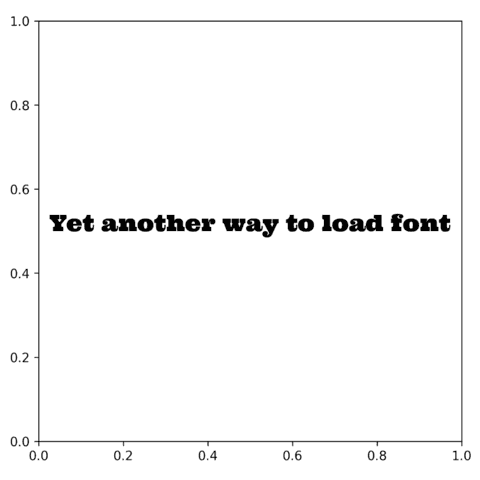
Load a locally stored font with pyfonts
import matplotlib.pyplot as plt
from pyfonts import load_font
font = load_font("path/to/myfont/Ultra-Regular.ttf")
fig, ax = plt.subplots(figsize=(6, 6), dpi=300)
ax.text(
x=0.5,
y=0.5,
s=f"Yet another way to load font",
font=font,
fontsize=18,
ha="center",
)
plt.show()
Gallery of examples
Here are some examples of what you can do with PyFonts
. Click on the images to see the code.
Going further
You might be interested in
- The official pyfonts documentation.
- The official github repo of pyfonts (⭐ give it a star!)
- Learn more on how matplotlib handle fonts
- How to draw arrows in matplotlib
- How to create beautiful annotation in matplotlib
🚨 Grab the Data To Viz poster!
Do you know all the chart types? Do you know which one you should pick? I made a decision tree that answers those questions. You can download it for free!
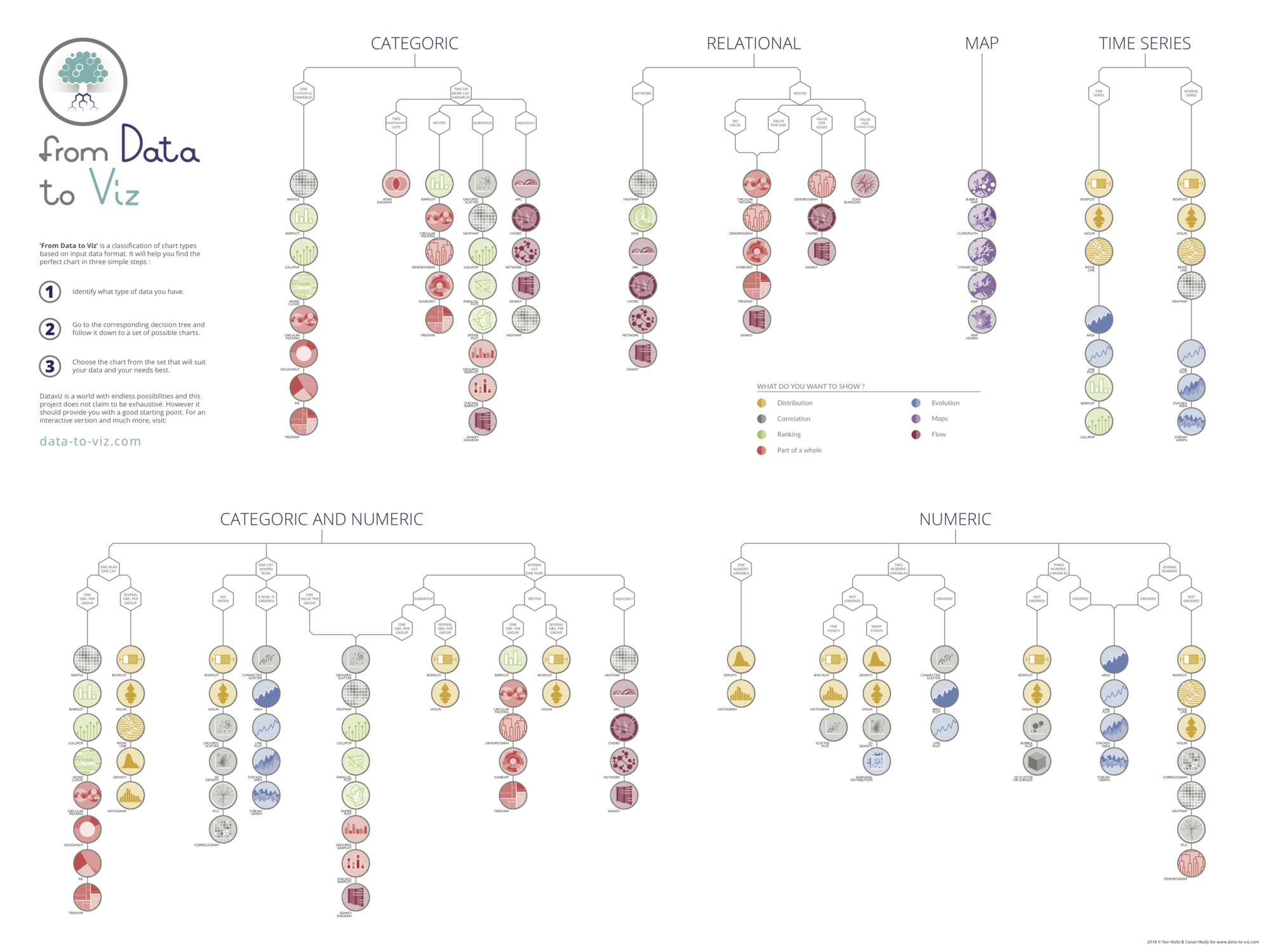